Of the crypto apps I have used, I’ve yet to find one that provides a Bitcoin price tracking widget to my liking. I’m not interested in the current price of Bitcoin, as I am interested in how my holdings (HODLINGS) are doing.
Thus, enter Scriptable, an automation app for Apple devices. It allows you to easily build widgets for iOS using good ol’ JavaScript. Additionally, the widgets can also be used on iPadOS and on your desktop machine running mac OS.
Previously, I’ve talked about Scriptable, back when I was originally learning how to do a layout with it. Eventually, those efforts made it into this particular widget.
To clarify, I don’t care much for the Bitcoin price. Undoubtedly, my interest is in how my own investment is doing. This type of widget seems pretty common with stock trading software, but not with cryptocurrency apps.
Generally, my needs from a Bitcoin price tracking widget are quite simple. Multiply how much Bitcoin (BTC) I own with the current price. Then deduct how much cash (United States Dollars) I’ve invested in said amount of Bitcoin to see how
Of those calculations, I’d like to see the total value of what I own, as well as my profit or loss. Not something I was interested in, but you could also take this a step further and also include the percentage of profit or loss.
Bitcoin price tracking widget
My approach to this was pretty simple. Hard code how much I invested and owned, ping the Coinbase API to grab the price, and then do some math. Altogether, the resulting widget, with some placeholder values, looks like this:
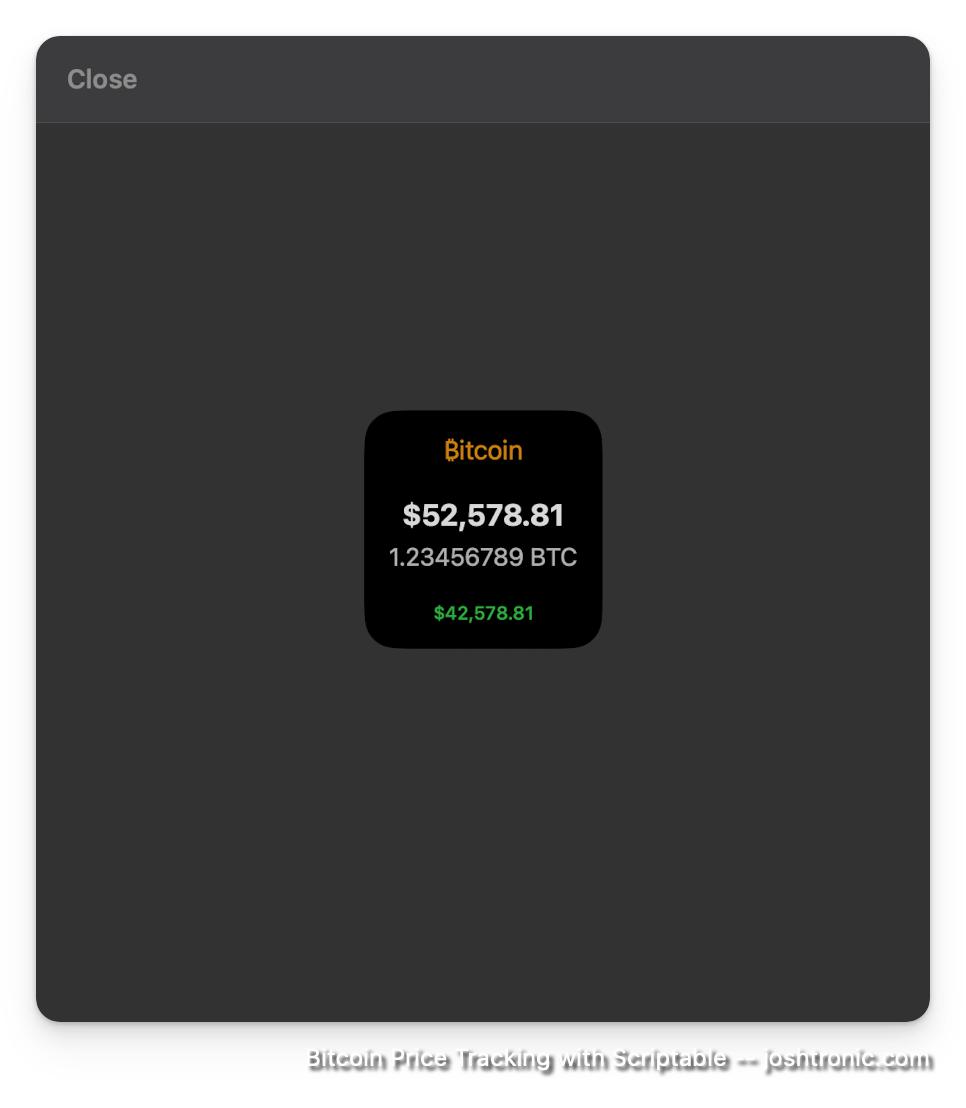
Scriptable code for the widget
While the code to make this happen isn’t anything to write home about, but it gets the job done. Obviously, you’ll want to adjust the variables invested
and owned
with your own values and you’re good to go:
const invested = 10_000.00;
const owned = 1.234_567_890;
const url = 'https://api.coinbase.com/v2/prices/BTC-USD/buy';
// Expected payload: {"data":{"base":"BTC","currency":"USD","amount":"22983.97"}}%
const request = new Request(url);
const response = await request.loadJSON();
async function createWidget(amount) {
const total = (owned * amount);
const widget = new ListWidget();
widget.backgroundColor = Color.black();
const layoutStack = widget.addStack();
layoutStack.layoutVertically();
const headerStack = layoutStack.addStack();
headerStack.addSpacer();
const titleEl = headerStack.addText('₿itcoin');
titleEl.textColor = Color.orange();
titleEl.textOpacity = 0.8;
headerStack.addSpacer();
layoutStack.addSpacer();
const contentStack = layoutStack.addStack();
contentStack.addSpacer();
const valueEl = contentStack.addText(`$${total.toFixed(2).toString().replace(/\B(?=(\d{3})+(?!\d))/g, ',')}`);
valueEl.font = Font.boldSystemFont(60);
valueEl.minimumScaleFactor = 0.1;
layoutStack.addSpacer(6);
const btcStack = layoutStack.addStack();
const btcEl = btcStack.addText(`${owned} BTC`);
btcEl.textOpacity = 0.8;
btcEl.minimumScaleFactor = 0.1;
contentStack.addSpacer();
layoutStack.addSpacer();
const footerStack = layoutStack.addStack();
footerStack.addSpacer();
const profitLossEl = footerStack.addText(`$${(total - invested).toFixed(2).toString().replace(/\B(?=(\d{3})+(?!\d))/g, ',')}`);
profitLossEl.font = Font.mediumSystemFont(12);
profitLossEl.textOpacity = 0.8;
if (total > invested) {
profitLossEl.textColor = Color.green();
} else {
profitLossEl.textColor = Color.red();
}
footerStack.addSpacer();
return widget;
}
const widget = await createWidget(response.data.amount);
if (config.runsInWidget) {
Script.setWidget(widget);
} else {
widget.presentSmall();
}
Script.complete();
JavaScript